You can authenticate a phone number by delivering a one-time password (OTP) via a phone call. To do this, you call the number and read a sequence of digits to the recipient via text-to-speech. To verify the number, the user needs to confirm the digits by entering them using the phone’s keypad.
Developers commonly use voice OTP to verify new user registrations, online transactions, and login sessions in an app or website. In this blog post, we walk you through a sample implementation of sending a voice OTP using the Plivo's Voice API and PHLO, our visual workflow builder. Plivo’s direct carrier connectivity and intelligent routing engine guarantee the best call connectivity and quality.
Prerequisites
Before you get started, you’ll need:
- A Plivo account — sign up for one for free if you don’t have one already.
- A voice-enabled Plivo phone number if you want to receive incoming calls. To search for and buy a number, go to Phone Numbers > Buy Numbers on the Plivo console.
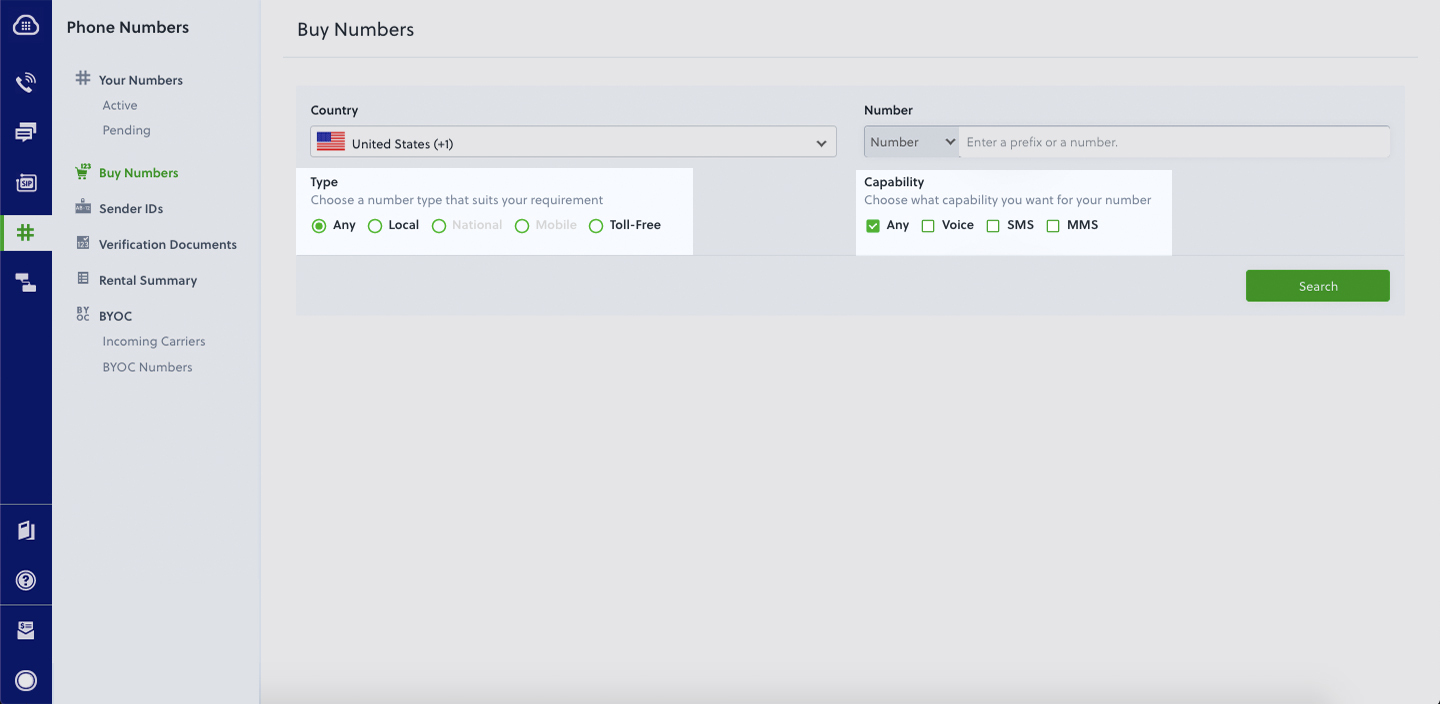
- ngrok — a utility that exposes your local development server to the internet over secure tunnels.
Create a PHLO to send OTP via phone call
PHLO lets you construct your entire use case and build and deploy workflows visually. With PHLO, you pay only for calls you make and receive, and building with PHLO is free.
To get started, visit PHLO in the Plivo console and click on Create New PHLO to build a new PHLO. On the Choose your use case window, click Build my own. The PHLO canvas will appear with the Start node. Click on the Start Node, and under API request, fill in the Keys as from, to, and otp, then click Validate. From the list of components on the left-hand side, drag and drop the Initial Call component onto the canvas and connect the Start node with the Initiate Call node using the API Request trigger state.
Configure the Initiate Call node with the using the From field and in the To field. Once you’ve configured a node, click Validate to save the configurations. Similarly, create a node for the Play Audio component and connect it to the Initiate Call node using the Answered trigger state. Next, configure the Play Audio node to play a specific message to the user — in this case, “Your verification code is <otp>.” Under Speak Text, click on Amazon Polly and paste the following:
Click Validate to save.
Connect the Initiate Call node with the Play Audio node using the Answered trigger state. After you complete the configuration, provide a friendly name for your PHLO and click Save.
Set up a .NET Framework app
In this section, we’ll walk you through how to set up a .NET Framework app in under five minutes and start handling voice OTP.
- Create an MVC web app.
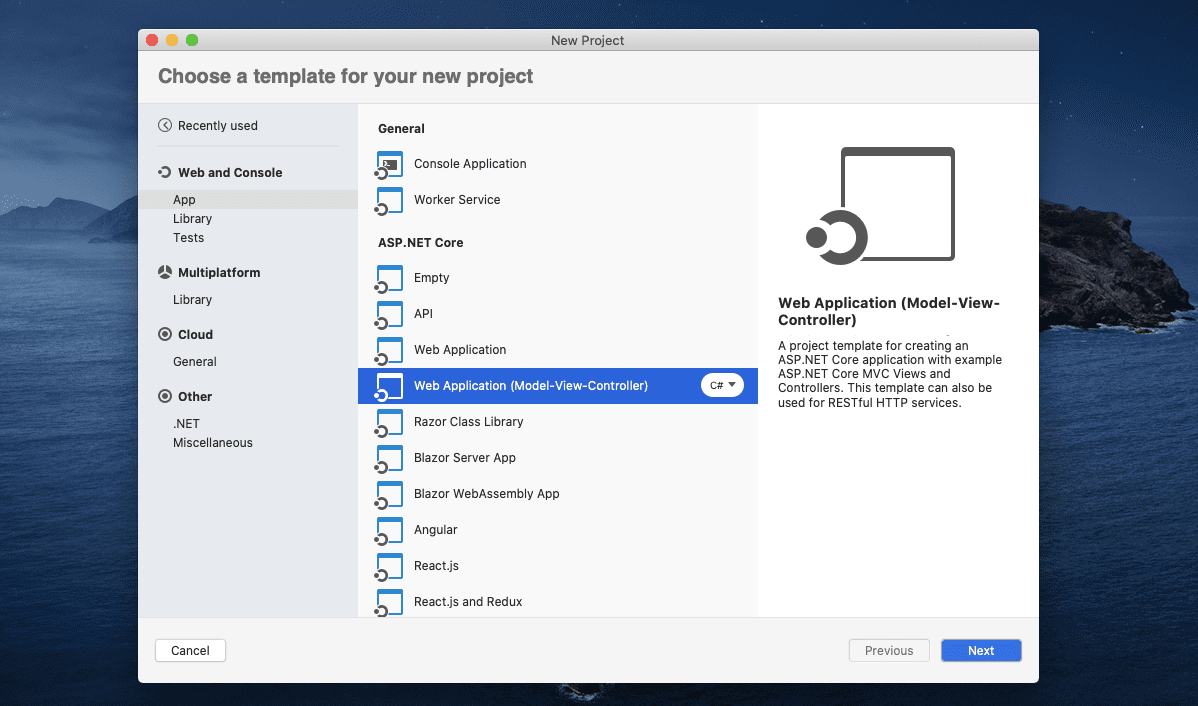
- Configure the MVC app and provide a project name.
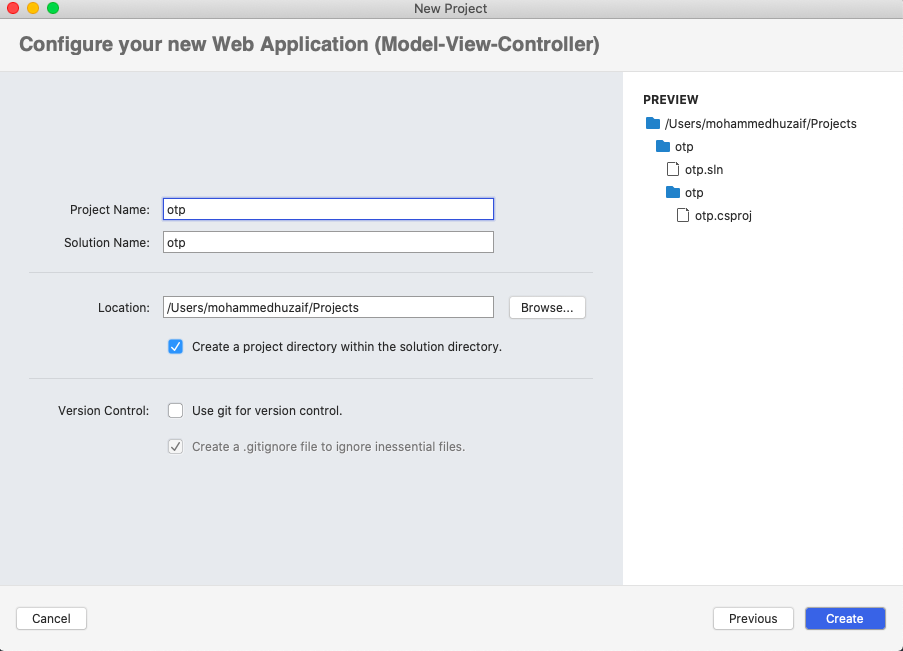
- Install the Plivo NuGet package.

- Install the Redis NuGet package.
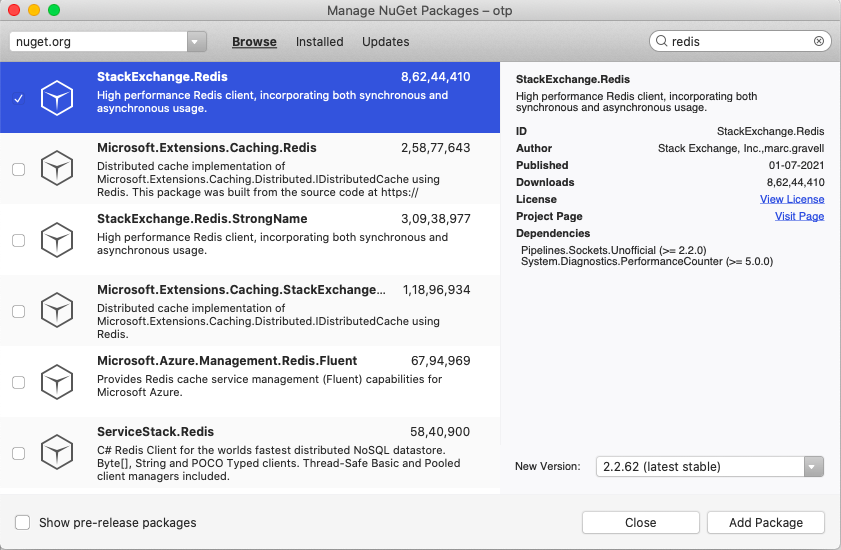
Trigger the PHLO
Once you’ve created and set up your .NET dev envrironment, go to the Plivo consolse and copy the PHLO_ID. You can integrate a PHLO into your application workflow by making an API request to trigger the PHLO with the required payload.
Navigate to the Controllers directory, create a Controller named otp.cs, and paste into it this code.
You can get your Auth ID and Auth Token from the console.

You can find the PHLO_ID on the PHLO Listing page.
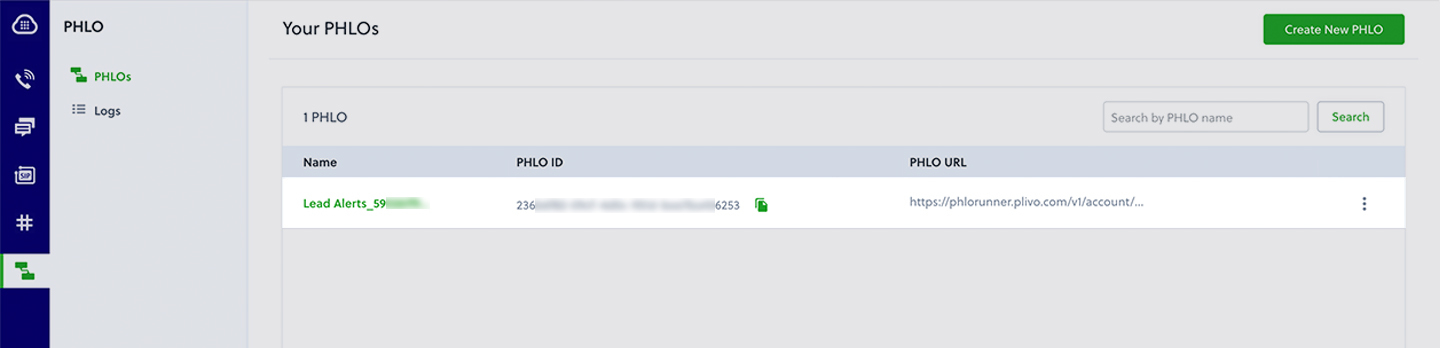
Test
Once you have created the Voice OTP app, save the file and run it.
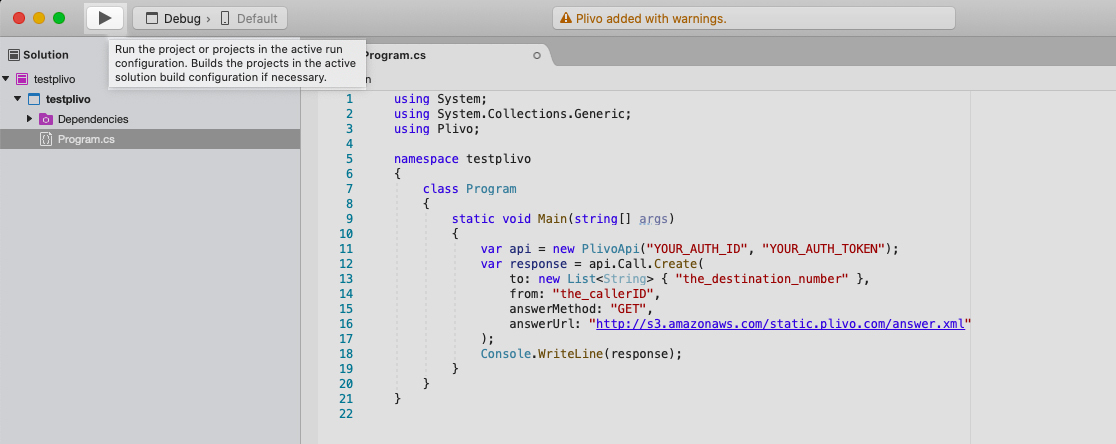
Start Redis.
$ redis-server
And you should see your basic server application in action.
https://localhost:5001/dispatch_otp/?destination_number=<destination_number>
https://localhost:5001/verify_otp/?destination_number=<destination_number>&otp=<otp>
Boom — you’ve made an outbound call with the OTP as a text-to-speech message.
Simple and reliable
And that’s all there is to send OTP via a phone call using Plivo’s Ruby SDK. Our simple APIs work in tandem with our comprehensive global network. You can also use Plivo’s premium direct routes that guarantee the highest possible delivery rates and the shortest possible delivery times for your 2FA SMS and voice messages. See for yourself — sign up for a free trial account.