Send messages globally with Plivo’s powerful SMS API Platform
Send and receive SMS globally with Plivo’s SMS APIs. Unlock lightning-fast SMS delivery to your customers across 220+ geographies.

Learn how Plivo powers billions of messages for global business giants
Plivo’s turnkey OTP solution
Verify API helps businesses authenticate new users and eliminate fake accounts
Unmatched performance, Unbeatable Pricing
Launch 10DLC Campaigns in 24 Hours!
We lead the industry in 10DLC innovation. Our automated registration process and unparalleled compliance support enable most campaign launches in under 24 hours. Say goodbye to manual headaches and hello to swift, compliant messaging.
Experience Plivo’s Pricing Advantage
We offer direct cost savings of up to 60% compared to leading competitors. Our transparent pricing model ensures you only pay for what you use, with automatic cost optimization features built-in.
Dynamic usage-based pricing automatically upgrades you to a better rate as your usage increases, allowing you to scale confidently.
Save more by auto-restricting delivery to non-SMS/MMS-enabled numbers.
Smart encoding reduces message lengths and leads to better savings.
Enjoy Plivo’s Global Coverage
Reach customers in 220+ geographies through direct connections with 1,600+ carriers. Our PoPs are located in seven locations (California, Virginia, Frankfurt, Mumbai, Singapore, Sydney, São Paulo) across five continents to ensure minimal latency and maximum impact on your campaigns.
Our internal infrastructure is hosted on a highly available cloud platform, guaranteeing 99.99% uptime.
We automatically re-route traffic to better-performing carriers to optimize message delivery rates.
World-class Customer Support At Your Fingertips
We are rated first in customer satisfaction. G2 users rate Plivo 99/100 for customer satisfaction, far above competing platforms. Don't settle for less when you can have the best!
Slash your support costs by 50%+ without sacrificing quality. Plivo's elite support plans deliver top-tier assistance at half the price of industry giants.
Expert, White-glove Onboarding
All new customers are greeted with white-glove onboarding assistance at no extra cost at each step of testing and integration.
Get personalized help from an expert team of solution engineers and sales personnel, followed by a customer success manager.
AI-powered Fraud Shield minimizes the risk of SMS pumping fraud
Plivo Fraud Shield is an AI-driven model that automatically detects and blocks fraudulent messages, saving over 95% of costs for your business.
Set up your SMS pumping fraud protection in just one click.
Unlock analytics and detailed reports for your account.
Integrate within just 2 mins!
Intuitive APIs and SDKs for a variety of programming languages like PHP, Python, Ruby, .NET, Nodejs, Java and Go - to make your integration a breeze. Our comprehensive documentation and sample code get you up and running in no time.
1import plivo
2
3client = plivo.RestClient('<auth_id>','<auth_token>')
4response = client.messages.create(
5 src='<from_number>',
6 dst='<destination_number>',
7 text='Hello, this is sample text',
8 url='https://<yourdomain>.com/sms_status/',
9 )
10print(response)
11#prints only the message_uuid
12print(response.message_uuid)
1require "plivo"
2include Plivo
3
4api = RestClient.new("<auth_id>","<auth_token>")
5response = api.messages.create(
6 src: "<from_number>",
7 dst:"<destination_number>",
8 text:"Hello, this is sample text",
9 url: "https://<yourdomain>.com/sms status/",
10)
11puts response
12#Prints only the message_uuid
13puts response.message_uuid
1var plivo = require('plivo');
2(function main() {
3 'use strict';
4 var client = new plivo.Client("<auth_id>", "<auth_token>");
5 client.messages.create(
6 {
7 src: "<from_number>",
8 dst: "<destination_number>",
9 text: "Hello, this is sample text",
10 url: "https://<yourdomain>.com/sms_status/"
11 }
12 ).then(function (response) {
13 console.log(response);
14 });
15})();
1package main
2
3import (
4 "fmt"
5
6 "github.com/plivo/plivo-go/v7"
7)
8
9func main() {
10 client, err := plivo.NewClient("<auth_id>", "<auth_token>", &plivo.ClientOptions{})
11 if err != nil {
12 fmt.Print("Error", err.Error())
13 return
14 }
15 response, err := client.Messages.Create(
16 plivo.MessageCreateParams{
17 Src: "<from_number>",
18 Dst: "<destination_number>",
19 Text: "Hello, this is sample text",
20 URL: "https://<yourdomain>.com/sms_status/",
21 },
22 )
23 if err != nil {
24 fmt.Print("Error", err.Error())
25 return
26 }
27 fmt.Printf("Response: %#v\n", response)
28 // Prints only the message_uuid
29 fmt.Printf("Response: %#v\n", response.MessageUUID)
30}
1<?php
2require 'vendor/autoload.php';
3use Plivo\RestClient;
4
5$client = new RestClient("<auth_id>","<auth_token>");
6$response = $client->messages->create(
7 [
8 "src" => "<from_number>",
9 "dst" => "<destination_number>",
10 "text" =>"Hello, this is sample text",
11 "url"=>"https://<yourdomain>.com/sms_status/"
12 ]
13);
14print_r($response);
15// Prints only the message_uuid
16print_r($response->getmessageUuid(0));
17?>
1import java.io.IOException;
2import java.net.URL;
3import java.util.Collections;
4
5import com.plivo.api.Plivo;
6import com.plivo.api.exceptions.PlivoRestException;
7import com.plivo.api.models.message.Message;
8import com.plivo.api.models.message.MessageCreateResponse;
9
10class MessageCreate
11{
12 public static void main(String [] args)
13 {
14 Plivo.init("<auth_id>","<auth_token>");
15 try
16 {
17 MessageCreateResponse response = Message.creator("<from_number>","+12025551111",
18 "Hello, this is a test message")
19 .url(new URL("https://<yourdomain>.com/sms_status/") )
20 .create();
21 System.out.println(response);
22 // Prints only the message_uuid
23 System.out.println(response.getMessageUuid());
24 }
25
26 catch (PlivoRestException | IOException e)
27 {
28 e.printStackTrace();
29 }
30 }
31}
1using System;
2using System.Collections.Generic;
3using Plivo;
4
5namespace PlivoExamples
6{
7 internal class Program
8 {
9 public static void Main(string[] args)
10 {
11 var api = new PlivoApi("<auth_id>","<auth_token>");
12 var response = api.Message.Create(
13 src: "<from_number>",
14 dst: "<destination_number>",
15 text: "Hello, this is sample text",
16 url: "https://<yourdomain>.com/sms_status/"
17 );
18 Console.WriteLine(response);
19 // Prints the message_uuid
20 Console.WriteLine(response.MessageUuid[0]);
21 }
22 }
23}
1curl -i --user auth_id:auth_token \
2 -H "Content-Type: application/json" \
3 -d '{"src": "<from_number>","dst": "+12025551111", "text": "Hello, this is sample text", "url":"https://<yourdomain>.com/sms_status/"}' \
4 https://api.plivo.com/v1/Account/{auth_id}/Message/
Flexible pricing model to fit your needs
Plivo provides a superior cost advantage over other major platforms.
Price Calculator

Only pay to verify REAL users
$0 Verification fee
$0 for Plivo Fraud Shield
Supercharge Your Business with Plivo's SMS Solutions
Two Factor Authentication
Dynamic verification codes with 95% conversion rate to secure your applications.
SMS Notifications
Deliver critical alerts, reminders etc. to 95% of recipients within 3 seconds.
SMS Marketing
Achieve a 98% open rate, with 90% of messages read within 3 minutes of delivery.
SMS Surveys
Gather insights 5x faster than traditional email surveys; average response time being 90sec
SMS Autoresponder
Handle up to 10,000 customer inquiries per hour, with 24/7 availability & zero wait times.
Appointment Reminders
Reduce no-show rates and increase overall appointment attendance.
Access powerful SMS features, including long message concatenation, support for any character set, delivery report notifications, MMS-rich media support, number pooling and local match, and more.
Prevent SMS Pumping from eroding your budget
Our SMS API has a built-in Plivo Fraud Shield - an AI-driven model that automatically detects and blocks fraudulent messages. Set up SMS pumping fraud protection in just one click.
Create a response to SMS pumping by choosing how your system responds to signs of SMS pumping fraud. Automate alerts to quickly take action in case of a breach.

Plivo by the numbers
Message delivery rate
Dispatch customized audio alerts using voice calls.
Message delivery time
Never miss sharing a critical reminder or update
Platform uptime
Experience the industry’s most reliable uptime rates.
Fraudulent activities detected
Fraud Shield analyzes and detects over 95% fraudulent activities.
Strategic Points of Presence
PoPs in California, Virginia, Frankfurt, Mumbai, Singapore, Sāo Paulo promise low latency communication.
Get the Plivo Advantage!
Plivo’s Key Differentiators
Plivo is a Trusted Partner for Superior Support, Guaranteed Delivery, and Simple Pricing
A simple solution to global messaging at scale
How do I start using WhatsApp messaging with Plivo?
Use our integrated sign-up and registration to start using WhatsApp messaging on Plivo’s console. Verify your WhatsApp numbers and begin sending messages within seconds.
How does the pricing work for using WhatsApp through Plivo?
Pricing is conversation-based, which includes Meta’s fees and Plivo’s pay-as-you-go platform fee. Meta charges per conversation, which is defined as a 24-hour message thread. Plivo charges a platform fee per conversation in addition to Meta's fees.
How does billing work with Plivo for WhatsApp messaging services?
Plivo consolidates all billing for both Plivo and Meta charges into a single invoice. This includes both the conversation-based charges by Meta and the platform fees by Plivo.
What our customers say about us?
Explore how Plivo empowers businesses to scale globally

Plivo’s Quick Guide to SMS Opt-In and Opt-Out
Research shows that most consumers want to receive text messages from brands they know and love. In fact, according to Yotpo, more than 80% of shoppers are already signed up to receive text messages from at least one brand.
Despite the data clearly indicating that your brand can and should take advantage of SMS marketing, you still explicitly need your customers' permission to text them. Opt-in and opt-out regulations dictate how individuals give or withhold consent for their personal information to be collected, used, or shared.
Opt-in and opt-out regulations differ from region to region. Some may mandate opt-in for certain data types, while others may allow opt-out. In this guide, we’ll outline the key tenets of opt-in rules, share the most widely applicable best practices, and suggest the best way to manage these permissions so your company remains compliant.
Why businesses must ask for opt-in permission
There are several reasons why businesses must ask their customers for opt-in permission. First and foremost, it’s a legal requirement in many places. For instance, the General Data Protection Regulation (GDPR) in the European Union mandates opt-in consent for certain types of data processing. Under GDPR, consent must be freely given, specific, informed, and unambiguous. Failing to obtain proper opt-in consent can lead to significant fines and legal issues for businesses.
Opt-in and opt-out options also help businesses demonstrate respect for user privacy and build customer trust. These permissions give users control over their personal information, which can strengthen a company’s reputation. Opt-in models require businesses to clearly explain:
- What data is being collected
- How it will be used
- Who it may be shared with
- The user's rights regarding their data
This ensures users make informed decisions about sharing their information.
Finally, and perhaps most importantly, opt-in/opt-out permissions minimize the risk of a privacy breach. By only processing data from customers who have explicitly granted permission to do so, companies can reduce their exposure to possible privacy violations.
What are the opt-in and opt-out requirements?
Opt-in and opt-out requirements describe someone’s actions concerning their personal data when accessing an app, website, or marketing campaign. For example, someone could choose to accept cookies, stop receiving text messages, or share their personal information to receive a newsletter.
Opt-in and opt-out requirements differ in their approach to obtaining consent from individuals regarding the collection and use of their personal data. Opt-in consent requires individuals to actively and explicitly agree before any processing occurs.
Opt-out requirements assume consent by default, meaning the user has to take action if they do not want their data collected or used. The table below shows a high-level comparison between opt-in/opt-out approaches.
There are certain legal requirements to be aware of as you set up your opt-in/opt-out collections process. GDPR isn’t the only regulation governing consumer privacy with data processing consent requirements. Many countries, including EU member states, Canada, and Australia, require opt-in consent for email marketing. The United States, under the CAN-SPAM Act, allows opt-out approaches for commercial emails.
Rules aren’t limited to governmental bodies, either. Gmail and Yahoo have also issued new email sender guidelines which require every email to have visible and accessible unsubscribe options.
Opt-in: a deeper dive
For businesses, the level of opt-in required varies based on the type of message being sent. The CTIA, a trade association for the U.S. wireless communications industry, outlines three main categories:
Promotional messages
Promotional messages require explicit written consent from customers before you can send them.
A promotional SMS marketing message encourages customers to take action, such as purchasing, attending an event, or engaging with a product. Examples include:
- Product recommendations
- Event announcements
- Seasonal offers
Informational messages
Informational messages require written or verbal permission from customers prior to sending.
An informational message is an update related to a customer's account or their relationship with the business. These messages include:
- Account notifications
- Loyalty program reminders
- Appointment confirmations
- Welcome messages
Conversational Messages
Conversational messages do not require explicit opt-in consent. These types of messages are one-on-one exchanges between a business and a customer, initiated by the customer. As such, the customer's initial outreach implies consent for that specific conversation. However, this doesn't grant permission for marketing messages or discussions on unrelated topics.
Here are some more specific opt-in and opt-out scenarios to consider.
Double opt-ins
A double opt-in is one in which a user signs up for an email marketing list or to receive SMS, and then a message (email or text) is sent to the user to click and confirm the subscription. The confirmation click is required for the user to receive future communications.
The double opt-in confirmation method not only helps reduce the risk of spam emails or fake numbers but it also reduces instances of bots signing up for your communications. This added step ensures that all your recipients are real people who genuinely want to hear from your company. This translates to higher engagement rates and lower costs.
Opt-in for websites, social media, and email
Opt-in approaches for websites, social media, and email share some common principles, but also have platform-specific requirements.
Websites must get explicit opt-in consent before using non-essential cookies. The easiest way to get this consent is to show a banner or pop-up when a user first enters the site. You may also want to include opt-in consent to use analytics tools, depending on your location.
Social media platforms also generally rely on opt-in consent for data collection and storage, but the platform itself will handle that for you. For example, a Facebook user agrees to Meta’s terms of service and privacy policies when creating an account. You don’t have to ask a second time when you view your business profile’s analytics.
However, some platforms may require additional opt-in consent for certain ad targeting or data-sharing scenarios. Facebook requires explicit opt-in for some sensitive ad categories.
Opt-in for email marketing and SMS
Email and SMS campaigns have their own set of rules that your business needs to know. You must get explicit consent before sending any marketing messages via email or SMS. For email, consent is required but can be implied in some cases (such as if the user has an existing business relationship).
SMS has one additional requirement. Your SMS messages must include clear disclosure of message frequency, potential carrier charges, and how to opt out if the user no longer wishes to receive messages from your business. Follow these best practices when designing your SMS opt-in approach:
- Keep initial messages short (under 160 characters)
- Clearly state your company name and purpose of messages
- Provide immediate value to the recipient (e.g. welcome offer)
- Make opting out easy with clear instructions
Your email campaigns must include your company’s physical address, as well as an unsubscribe mechanism. Like SMS, try to make the sign-up process simple and user-friendly.
Offer a preference center to manage subscriptions and set clear expectations about email content and frequency.
How to incentivize opt-ins
Now that you know the guardrails governing opt-ins, how can you encourage your audience to sign up for and continue receiving your SMS marketing?
First and foremost, as the saying goes, you have to give something to get something. Give your audience a clear reason for subscribing to your SMS messaging list, such as a discount, early access to sales, or personalized shopping recommendations. In the process, set clear expectations: let the customer know how often they can expect to hear from you, as well as what kind of texts they will be receiving (for instance, order updates, appointment reminders, or something else).
Next, make it easy to say yes. Use keywords for easy text-to-join options; for example, a pet supplies subscription box could ask customers to text “WOOF123” to a short code used by the brand. Any forms that need to be filled out should be optimized for mobile. Think about your sign-up process, too.
Finally, to retain customers, make sure you stick to your cadence and continue offering value in your SMS marketing outreach. If you start to see opt-out rates creep up, check on your analytics to make sure there are no issues with your delivery. Then, consider whether your SMS messaging delivers your audience something unique and consistent with the rest of your marketing efforts.
Opt-in and opt-out best practices
Crafting effective opt-in prompts is more than just a formality. It's a strategic decision that can foster a loyal, engaged audience. By adhering to best practices, you'll enhance your customer experience, ensure compliance with data privacy laws, and build trust. Follow these best practices to make it easy for your audience to control how much they share with your business.
- Don’t pre-check the opt-in box. Some forms are set up so that the user has to opt out and uncheck a box to avoid receiving emails. It’s a sneaky way for a brand to collect user information since most people don’t realize they must uncheck the box. Clearly, this does not foster trust with your audience.
- Use a CAPTCHA or OTP to secure your sign-up form. A CAPTCHA can help prevent bots from signing up for your SMS list. Screen out bad actors to prevent cost overruns and protect your subscriber list.
- Maintain your list regularly. Update your contact lists regularly to remove unresponsive subscribers and invalid numbers. Cleaning up your list ensures you are only messaging with engaged users and not spending your budget sending SMS to those who won’t respond.
- Use double opt-in. Employing a double opt-in approach can significantly improve your campaign results. When users opt-in twice, you can be assured that they definitely want to hear from and engage with your brand. This leads to better quality and higher performance.
- Make your opt-in message crystal clear. Your SMS opt-in form must specifically mention SMS as the communication channel. In the US, 10DLC compliance mandates that you mention SMS in your opt-in form. For example, your opt-in form could read, “Check this box to opt-in to receive SMS text messages from (Your Company Name). Standard rates and data may apply. Reply STOP to opt out."
Ultimately, the goal is clear, simple communication with your SMS subscriber list. These tools can help you manage opt-in to achieve that outcome.
How to manage opt-in and opt-out requests
Tools like Plivo make it easy to manage opt-in and opt-out requests. Plivo’s SMS platform automatically handles SMS opt-out if your contact replies with an opt-out keyword. We support these opt-out keywords:
- Stop
- Cancel
- End
- Quit
- Unsubscribe
Plivo also supports 10DLC opt-in consent through voice, web form, keyword, and even paper form. We provide extensive guidance on how to collect opt-in consent in our developer documentation. We strongly recommend consulting our A2P opt-in best practices before launching your campaign.
Conclusion
Plivo’s SMS API is one of the most effective ways to engage with customers worldwide. With SMS coverage in over 220 countries across North America, Europe, Asia, Oceania, South America, and Africa, Plivo customers use opt-in daily to maintain and grow their contact lists. Powerful, out-of-the-box features beyond opt-out automation help your business gain a competitive advantage in SMS communications.
Learn more about Plivo’s platform and how our API could work for your business. Get in touch to request a trial.
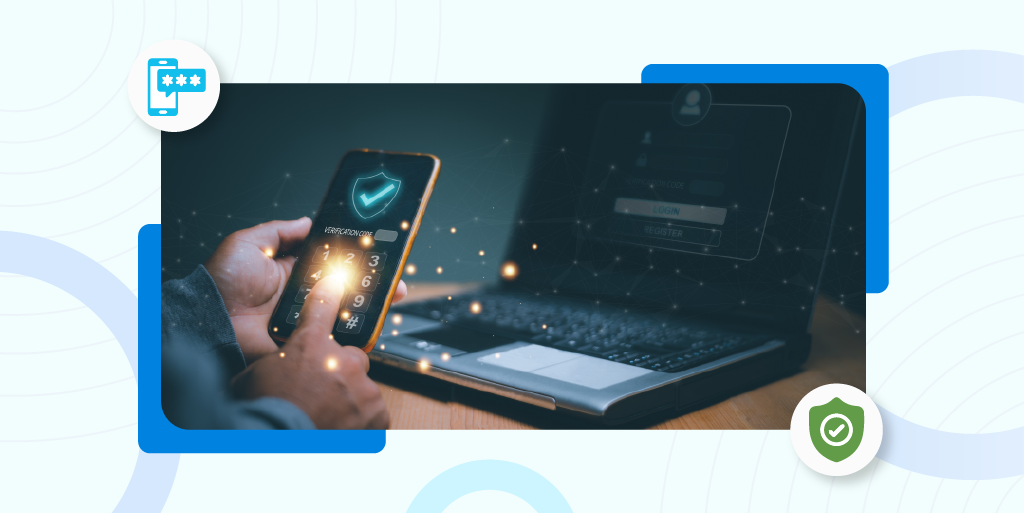
Why SMS / Voice Verification is Here to Stay
Okta will sunset its SMS and voice verification service on September 15, meaning all Okta users need to bring their own provider (BYOP) to continue offering one-time passcode (OTP) verification using these channels. Okta will focus on password-less options like FastPass or FIDO2 WebAuthn.
While FastPass and WebAuthn undeniably offer advanced security features, we believe SMS and voice authentication methods remain relevant in enterprise environments. There are compelling reasons enterprises should continue using Plivo with Okta for SMS and voice OTP authentication. Not only that, but Plivo makes it easy to integrate with Okta and make sure your customers never experience a delay, miss an OTP, or get frustrated trying to log in.
{{cta-style-1}}
Here are some key benefits and considerations that make these channels both a viable and valuable choice for businesses today.
6 SMS and voice OTP advantages
1. Universal accessibility and compatibility
The wide user reach makes SMS and voice verification a good option for global enterprises. SMS and voice authentication methods are not limited by the type of device a user has. Whether someone is using a basic feature phone or a high-end smartphone, SMS and voice work seamlessly across all mobile devices. Users don’t need to install specific applications, possess hardware tokens, or have smartphones.
Using multiple channels for OTPs also creates redundancy that fosters greater reliability. SMS and Voice OTPs serve as reliable backup methods when primary authentication methods fail or are unavailable due to technical issues or user device problems. This redundancy ensures continuity and reduces the risk of access interruptions.
Did you know? Plivo also supports WhatsApp, with email and RCS messaging coming soon!
This inclusivity ensures users can participate in secure authentication processes regardless of their technological capabilities or resources.
2. Ease of integration
On the business side, most enterprises already support SMS and voice OTPs, making these methods easy to maintain and expand. This established infrastructure reduces the need for significant investment in new systems, allowing businesses to continue leveraging their existing resources. Plivo’s Verify API integrates seamlessly, allowing developers to slash implementation time by 90%.
3. User familiarity and convenience
Because OTPs enjoy global reach, the process is straightforward and familiar to most users. The simplicity of receiving and entering a code into a system makes SMS and voice OTPs convenient for users of all ages and technical proficiency levels. This familiarity reduces the need for extensive training and support, enabling smoother adoption and fewer usability issues.
For developer teams, unlike advanced authentication methods that may require setup, enrollment, or understanding of new technologies, SMS and voice authentication can be deployed immediately. Plivo’s Verify API is ready to go in just one sprint.
4. Support for non-corporate users
Not all users interacting with an enterprise's systems have corporate-managed devices or can install apps like FastPass or use WebAuthn’s advanced features. Contractors, remote workers, and temporary workers still need a way to secure their accounts without requiring corporate IT involvement. OTPs allow for secure authentication without complex device management policies, making them ideal for BYOD and hybrid work scenarios.
5. Affordable and scalable
OTPs have low initial investment costs: enterprises do not need to purchase and distribute hardware tokens or ensure all users have compatible devices. This reduces the upfront costs associated with more advanced authentication methods.
SMS and Voice services can be easily scaled up or down based on demand, allowing businesses to adjust their authentication capabilities without significant additional costs or logistical challenges. Plivo offers flexible pricing models, enabling organizations of all sizes to adopt these methods economically. Plus, Plivo Verify comes with:
- Zero authentication fees
- Fraud Shield for free
- No regulatory overhead
- No need to purchase numbers
- Reduced technical support costs
- No monthly phone number rental fee
Supporting advanced authentication methods can increase technical support needs due to issues like setup, device compatibility, or app installations. SMS and voice-based methods, however, are simple and intuitive, requiring little to no user guidance and reducing the burden on IT support.
6. Meet compliance standards
In some industries, such as finance and healthcare, and in certain regions, SMS and voice OTPs are recognized and accepted methods for multi-factor authentication. These methods help enterprises meet regulatory requirements without the need to adopt newer technologies that may not yet be standardized.
Plivo is HIPAA, GDPR, and PCI DSS compliant, with SOC 2 and ISO 27001:2022 certification.
Get started with Plivo
While modern authentication methods like FastPass and WebAuthn offer enhanced security, SMS and voice OTPs remain relevant and valuable for all businesses. Their universal accessibility, ease of use, cost-effectiveness, and role as a reliable backup make them indispensable in various enterprise contexts.
Okta users can integrate Plivo in five minutes or less. Our off-the-shelf API is designed to “go live in one sprint.” With a 95% OTP conversion rate and the lowest costs per conversion, Plivo’s Verify API is well suited for businesses of all sizes. Learn more to ensure secure, inclusive, and resilient access for all users, regardless of their technological capabilities.

5 Best SMS API Providers to Consider in 2024
SMS isn't just a nice-to-have; today, it's the backbone of great customer experiences.
In fact, your SMS strategy may impact your brand reputation more than you realize. More than 60% of consumers say that businesses that text them provide a better overall customer experience than companies that don’t.
Surprisingly, most consumers receive texts from one to three businesses; but they’re willing to opt into texts from up to six businesses.
There’s still untapped potential to be mined from SMS marketing. And, the easiest way to gain a competitive advantage is with an SMS API provider.
Choosing the best SMS API provider is about reliability, cost, and, ultimately, finding the perfect fit for your business. Your SMS API provider should help you connect deeper with your customers, scale effortlessly, and future-proof your business.
In this guide, we're diving deep into what to look for in an SMS API provider and sharing the best bulk SMS API providers in the market in 2024. Read on to find your perfect match and start optimizing your SMS marketing efforts.
What to look for in an SMS API provider
Trust is everything when it comes to texting customers. Your SMS API provider should help you stay compliant and communicate in a way that makes your customers feel secure giving you their personal contact details. A 2021 Deloitte study shows that, on average, consumers spend 25% more money on trusted brands.
Look for SMS API providers that make it easy to follow industry regulations, among other criteria, so that you can reliably reach your customers without putting their information at risk.
{{cta-style-1}}
Reliability and uptime
The best SMS gateway API provider delivers your messages on time, every time. This should be the baseline standard for any API you consider. Need to confirm appointments or update customers on deliveries? You should be able to send an SMS and never think twice. For instance, Plivo's 99.99% uptime guarantee ensures that your time-sensitive messages are delivered reliably, letting you send messages in total confidence.
Pricing and value for money
Most businesses will consider price first and foremost when selecting a provider. And while the price tag is obviously a huge factor, consider the bigger picture. What value are you getting from your provider? The lowest-priced option doesn’t guarantee high-quality service, security, or reliability.
Your pricing assessment should factor in volume discounts, pricing models, and hidden fees. You want the best value, and that’s where SMS API providers like Plivo shine. We offer competitive pricing and scalable solutions.
Geographic coverage
Wherever your customers are today, your SMS API provider must be able to reach them. You may not need to send messages worldwide in your current operational capacity. But, if your business is still growing, it might be nice to have the flexibility to deliver your messages wherever your customers live and work.
Features and customization options
The best SMS API providers let you personalize messages, track results, and build stronger customer relationships. It's not just about sending texts; it's about creating meaningful connections.
Ease of integration and documentation
Developers already have a lot on their plate. Save them time by opting for an API with clear and comprehensive documentation and easy integration. It’s a win-win for your team and your customers!
Customer support
Look for an SMS API provider that is available 24/7. This level of service translates to immediate assistance, minimized downtime, and enhanced reliability. Your company can also benefit from the convenience of multiple support channels, such as email, phone, web-based chatbots, and SMS.
5 best SMS API providers: at a glance
Need help picking the right SMS API provider for your company? We've compared five of the most popular providers, breaking down their features, pros, cons, and pricing. Check out our in-depth analysis following the table below to find the best fit for your business.
1. Plivo
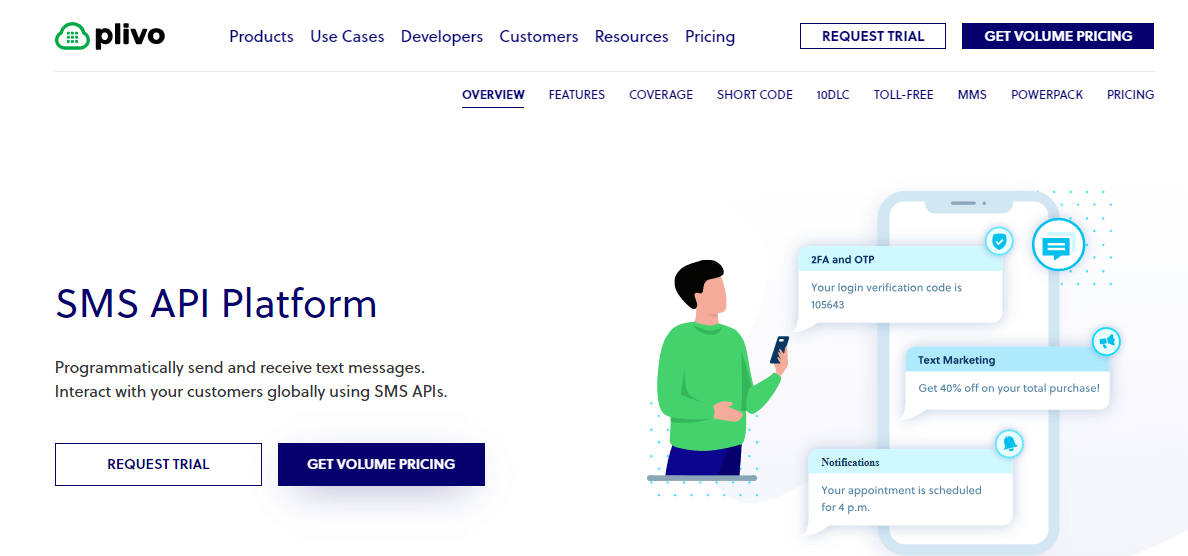
Key features
With Plivo, you can focus on growing your business, not troubleshooting your tech. The Plivo SMS API handles the heavy lifting of scaling globally, with features such as:
- Smart queuing: Plivo’s smart queuing feature allows you to send multiple SMS messages in a single API request. Plivo dynamically manages message priority and expiry, handling message queueing for you.
- Built-in fraud control: Protect your business from SMS pumping fraud with Plivo's Fraud Shield. Its geo permissions feature lets you control the countries to which your SMS traffic is sent, while the fraud thresholds feature sets limits on message volume and adds an extra layer of security to your SMS strategy — at no extra cost.
- 24/7 customer support: We offer a range of support plans to meet any organization’s needs, from a free basic plan to a 24/7 premium support option. No matter your plan, you’ll have access to our team of experienced support engineers. Premium support plan users, however, get prioritized responses to their queries within a guaranteed timeframe, depending on the priority of the issue.
- Message redaction: Plivo's message redaction feature can hide sensitive details like numbers and names while still getting the message across if you need to send sensitive information via text. This makes perfect sense for highly regulated industries, such as healthcare, financial services, and education. Implementing redaction with two-factor authentication (2FA) increases overall security and ensures compliance
- Multi-language messaging support: Plivo’s built-in GSM Unicode character set lets your users communicate in any language, including Chinese, Japanese, and Arabic, and with symbols and emojis.
- MMS-rich media support: You can send and receive photos, videos, and audio clips, as well as picture messages and SMS texts using the same phone number.
- Real-time delivery notifications: Get real-time delivery notifications of messages sent globally. Track delivery rates and account performance.
- Global compliance: Non-compliance with regional security protocols increases the risk of hefty fines and puts your reputation at risk. Plivo’s SMS API adheres to security best practices, including 2FA, to help protect your communications.
Pros
- High API call speed
- Reliable service and uptime
- Privacy Shield and GDPR compliance
- Support for any character set
- Easy to integrate with comprehensive documentation and sample codes
- Server-side SDKs in all popular programming languages
- Ready-to-use templates to add functionalities
- Usage-based pricing with additional volume discounts
Cons
- A steep learning curve for new users
Pricing
Offers a pay-as-you-go pricing model
Suitable for
Most suitable for companies that need a scalable, customizable SMS API provider with extensive integration capabilities, security, and reliability
Reviews and ratings
G2: 4.5 stars ( 724 reviews)
{{cta-style-1}}
2. Twilio

Key features
- Supports MMS: You can drive two-way engagement with cross-channel messaging for MMS and more using a single API.
- Screen-sharing and live chat: Developers can add live chat and share screens. Plus, they can create their own custom video players.
- Personalized messages: You can customize messages for reminder notifications, delivery updates, and more.
Pros
- Easy integration
- Handles high volumes of messages
- High deliverability
Cons
- A steep learning curve for new users
- It gets expensive with usage
Pricing
The SMS/MMS pricing differs for each country; go through Twilio’s official website for all pricing plans.
Suitable for
Companies across industries seeking a comprehensive, scalable, and programmable messaging solution.
Reviews and ratings
G2: 4.2 stars (471 reviews)
Note: Many reviewers on Quora highlight Twilio for its reliability and features. However, others report that Twilio can become costly with high usage and may have scalability issues. For those seeking a cost-effective and reliable messaging solution, Plivo is a top Twilio alternative.
3. Messente

Key features
- Message templates: Messente creates and uses message templates for different scenarios, such as transactional updates, promotional messages, or alerts.
- Schedule messages: You can schedule messages for future delivery and automate sending based on specific triggers or events.
Pros
- Easy to integrate
- Solid customer support
- User-friendly interface
Cons
- Expensive for bulk messages
- There may be delays in sending SMS due to the reliability of network partners
Pricing
It starts at 0.01 €(0.011 $) per message. Reach out to their sales team for custom pricing.
Suitable for
Companies seeking a reliable global messaging solution with strong customer support.
Reviews and ratings
G2: 5 stars (2 reviews)
4. Telnyx

Key features
- Automatic opt-outs: If a customer responds to their message with "stop," it'll automatically unsubscribe them from future communication.
- Message concatenation: The API automatically breaks content into different sentences if the message is too long for its destination.
- Intelligent text encoding: The Telnyx system automatically chooses the most compact encoding possible, minimizing the cost per send.
Pros
- 24/7 customer support
- Offers competitive pricing
- Scalable API
Cons
- Lacks advanced features
- Not as reliable as other providers on this list
Pricing
Starts at 0.004$ per message. Reach out to their sales team for custom pricing.
Suitable for
Companies on a modest budget seeking a comprehensive messaging solution.
Reviews and ratings
G2: 4.7 stars (405 reviews)
5. Bird
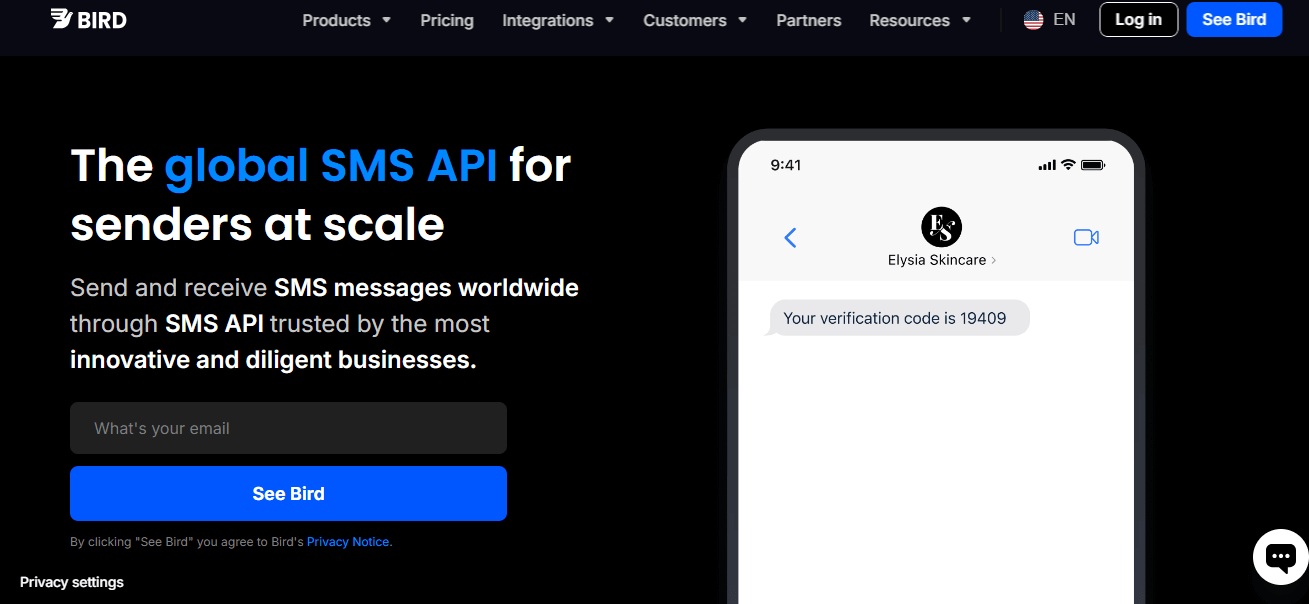
Key features
- Multi-channel support: MessageBird supports channels like WhatsApp, SMS, chatbot, SIP Trunk, and Google Business Messages.
- Interactive SMS features: It supports interactive SMS features such as SMS surveys and polls, enabling more dynamic and engaging communication with recipients.
Pros
- High-speed API calls for fast communications
- Provides clear, effective API documentation
- Carrier-level analytics
Cons
- Gets expensive with usage
- API may take time to load
Pricing
Starts at $45 per month. Reach out to their sales team for custom pricing.
Suitable for
Companies seeking a messaging platform to engage customers and collect feedback through SMS.
Reviews and ratings
G2: 4.1 stars (67 reviews)
Choosing the right bulk SMS API provider for your business needs
Choosing the best SMS API provider for your business is no easy task. Here’s a recap of what to look for as you evaluate possible SMS API partners.
1. Align API capabilities with business requirements
Choosing the right SMS API provider is a different process for every business. There’s no one-size-fits-all solution; however, Plivo’s customers appreciate its reliability, global reach, ease of use, responsive support, and competitive pricing.
2. Assess scalability and future growth
Select an SMS API provider that can grow with your business. Evaluate the API’s ability to handle increasing message volumes, geographic expansion, and evolving communication needs. Scalable APIs, like those offered by Plivo, support high-traffic demands and offer flexible pricing, ensuring they remain effective as your business scales and diversifies.
3. Ensure compliance and data security
Prioritize SMS APIs that adhere to regulatory standards with robust data security measures. Compliance with regulations such as TCPA, GDPR, and HIPAA is crucial for safeguarding user data and avoiding legal issues.
Choose SMS APIs like Plivo with built-in security features and clear policies on data protection to maintain trust and ensure your messaging practices are secure and compliant.
Plivo: The Best SMS API provider
Plivo stands out as the best SMS API provider for virtually any business due to its comprehensive features. Plivo users love the platform’s robust messaging capabilities, high deliverability and high reliability (up to 99.99% uptime SLAs), competitive pricing, and advanced analytics. Offering a user-friendly interface, Plivo can be integrated with multiple popular tools like Zapier.
Want to figure out if Plivo's the right choice for your needs? Request a trial and see how it elevates your SMS strategy.
Get started with Plivo today
Don't settle for overpriced, underperforming SMS solutions. Experience the Plivo difference with our unbeatable combination of global reach, superior reliability, and cost-effective pricing.