Voice OTP is used to authenticate a phone number by delivering a one-time password (OTP) via a phone call. This verification is done by making a call to the mobile number and playing a sequence of digits. To verify the mobile number, the user needs to confirm the played sequence of digits.
OTPs are commonly used to verify new user registrations, online transactions, and login sessions in an app or website. In this blog post, we walk you through a sample implementation of sending a voice OTP using the Plivo Voice platform and PHLO, our visual workflow builder. Plivo’s direct carrier connectivity and intelligent routing engine guarantee the best call connectivity and quality.
Prerequisites
Before you get started, you’ll need:
- A Plivo account — sign up for one for free if you don’t have one already.
- A voice-enabled Plivo phone number if you want to receive incoming calls. To search for and buy a number, go to Phone Numbers > Buy Numbers on the Plivo console.
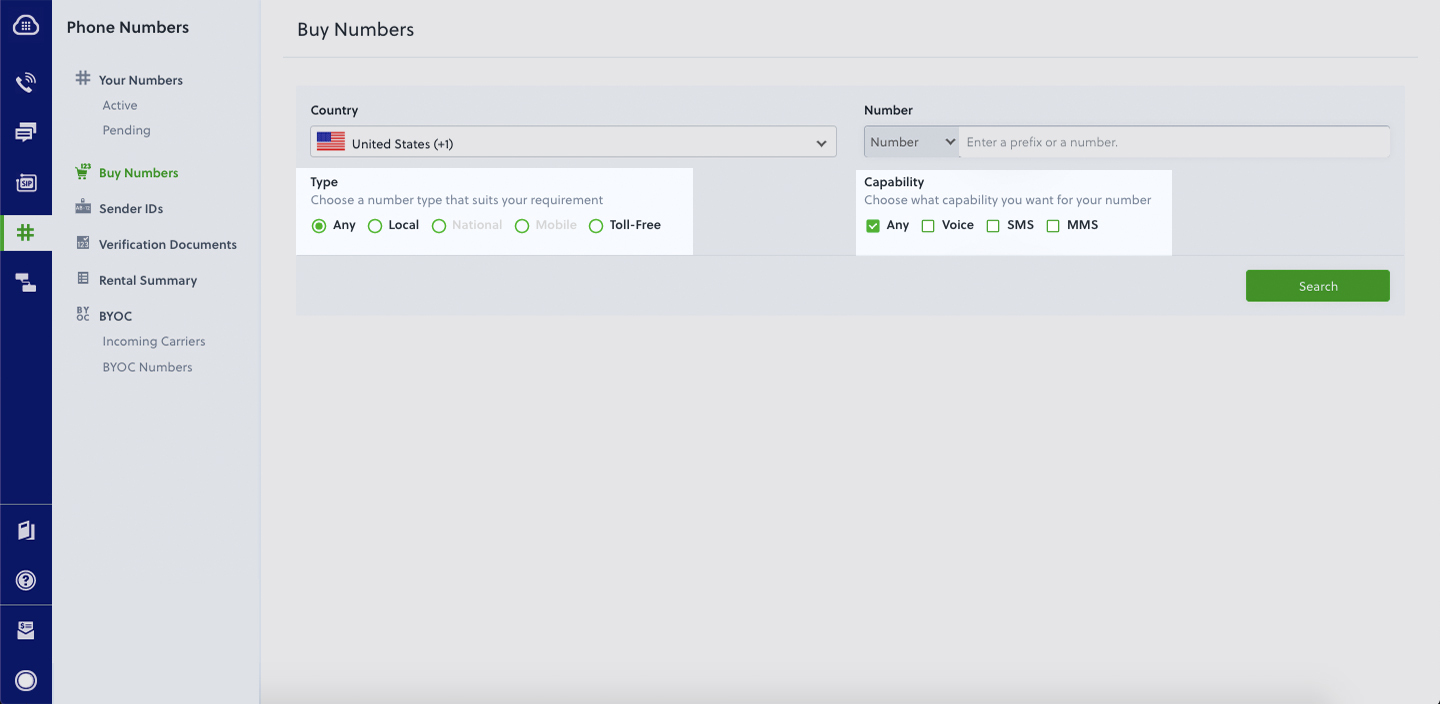
- Flask and Plivo Python packages — run pip install plivo flask to install them.
- ngrok — a utility that exposes your local development server to the internet over secure tunnels.
Create a PHLO to send OTP via phone call
PHLO lets you construct your entire use case and build and deploy workflows visually. With PHLO, you pay only for calls you make and receive, and building with PHLO is free.
To get started, visit PHLO in the Plivo console and click on Create New PHLO to build a new PHLO. In the Choose your use case window, click Build my own. The PHLO canvas will appear with the Start node. Click on the Start Node, under API request, fill in the Keys as from, to, and otp, then click Validate. From the list of components on the left side, drag and drop the Initial Call component onto the canvas and connect the Start node with the Initiate Call node, using the API Request trigger state.
Configure the Initiate Call node with the using the From field, and in the To field. Once you’ve configured a node, click Validate to save the configurations.
Similarly, create a node for the Play Audio component and connect it to the Initiate Call node using the Answered trigger state. Configure the Play Audio node to play a specific message to the user — for example, in this case, “Your verification code is <OTP>”. Under Speak Text, click on Amazon Polly and paste the following:
Click Validate to save.
Connect the Initiate Call node with the Play Audio node using the Answered trigger state.
After you complete configuration, provide a friendly name for your PHLO and click Save.
Use the PHLO in a Flask application
Now you can use the PHLO in a Python Flask application.
- Create a project directory and change into it.
- Install the Plivo SDK using pip.
- Install other modules.
We recommend that you use virtualenv to manage and segregate your Python environments, instead of using sudo with your commands and overwriting dependencies.
Run the PHLO to send OTP via phone call
Now you can trigger the PHLO and test it out. Copy the PHLO ID from the end of the URL of the workflow you just created. You’re also going to need your Auth ID and Auth Token. Create a Python source code file — let’s call it trigger_phlo.py — and paste this code into it:
Substitute actual values for <auth_id>, <auth_token>, and <PHLO_ID>. Save the file and run it.
Boom — you’ve made an outbound call with the OTP as a text-to-speech message.
Simple and reliable
And that’s all there is to send OTP via a phone call using Plivo’s Python SDK. Our simple APIs work in tandem with our comprehensive global network. You can also use Plivo’s premium direct routes that guarantee the highest possible delivery rates and the shortest possible delivery times for your 2FA SMS and voice messages. See for yourself — sign up for a free trial account.